How to: Develop plugins with CDS for Code
Table of Contents
Prerequisites
Developing plugins
Create a new plugin from scratch using Visual Studio
There is documentation here about creating and registering plugins in Visual Studio for use in CDS.
Create a new plugin from .NET Core CLI
In a new PowerShell or CMD window:
# Create the new project
dotnet new classlib -n MyPluginSample [Enter]
# Enter project directory
cd MyPluginSample [Enter]
# Create a new solution file
dotnet new sln [Enter]
# Add the project to the solution
dotnet sln add MyPluginSample.csproj [Enter]
We now have to sign the assembly. This can be done using the Sn.exe Strong Name Tool.
In the Visual Studio Developer command prompt:
sn -k MyPluginSample.snk
Next locate the MyPluginSample.csproj file and replace it’s contents with the following XML
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>net452</TargetFramework>
<AssemblyVersion>1.0.0.0</AssemblyVersion>
<FileVersion>1.0.0.0</FileVersion>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Microsoft.CrmSdk.CoreAssemblies" Version="8.2.0.2"/>
</ItemGroup>
</Project>
Next locate the Class1.cs and rename it to FollowupPlugin.cs.
Then replace it’s file contents with the following code:
using System;
using Microsoft.Xrm.Sdk;
namespace MyPluginSample
{
public class FollowupPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
throw new NotImplementedException();
}
}
}
Now your project is created and assembly is signed. To see the rest of the code you can finish the rest of the tutorial found here.
Create a new plugin from a template
Note: A major advantage to building plugins using templates is that the CloudSmith Plugin Template comes with built in helpers that can make developeing plugins faster with less code.
Within VSCode File Explorer
- Right-click the directory you want the project parent folder created within
- Click New Templated Project
- Enter the project namespace and assembly name then press enter
- Enter the class name for the project then press enter
Within CDS for Code Template Explorer
- Expand Project Templates
- Expand CloudSmith Consulting LLC
- Right-click the target template to use
- Click Create in workspace
- Select the directory you want the project parent folder created within, or create a new one
- Enter the project namespace and assembly name then press enter
- Enter the class name for the project then press enter
You’ll find the CDS for Code Template Explorer in the VSCode Explorer view. It will look similar to this:
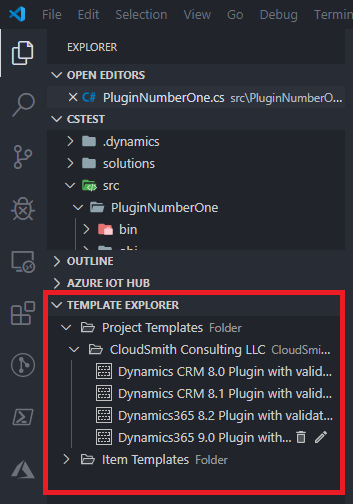
Note: Once your project is created you may see a VSCode notification saying that the project needs packages to be restored. Saying yes will restore the packages used by the plugin project.
Use an existing plugin project from Visual Studio
Coming soon
Registering plugins
Deploy from File Explorer
Plugins can be deployed through the context menu of *.csproj project files in VSCode File Explorer.
- Right-click the target *.csproj file
- Click Build project
- Select if you want to see the logs from the build
- Right-click the target *.csproj file again
- Click Deploy assembly to CDS
- Select which DLL file you want to deploy (we recommend from the /bin folder)
- Select the solution you want the plugin assembly to be deployed within
- You may be prompted to select which assembly you want to overwrite
- If this is a new assebly simply press the esc key
- If you want to overwrite an assembly, select the assebly to overwrite then press enter
After the assembly is deployed to the server, the Configure Plugin Step dialog will be displayed. More about this can be found in the Plugin step UI section
Update/deploy from CDS Explorer
Coming soon
Managing plugin steps
Create plugin steps from CDS Explorer
Plugin steps can be created in CDS Explorer by:
- Open the CDS Explorer view by clicking the CDS for Code icon
- Expand the top level connection
- Expand the Organization
- Expand the Solutions folder
- Expand the target solution
- Expand the plugins folder
- Expand the target plugin assembly
- Mouse over the target plugin assembly namespace and click the plus icon
Your CDS Explorer view will look similar to this:
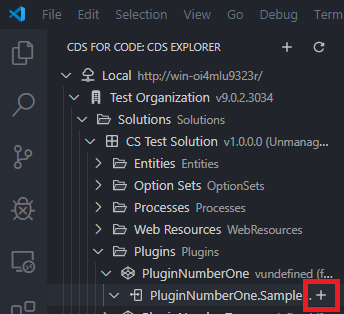
Plugin step UI
Creating or editing a plugin step will be done on the following screen:
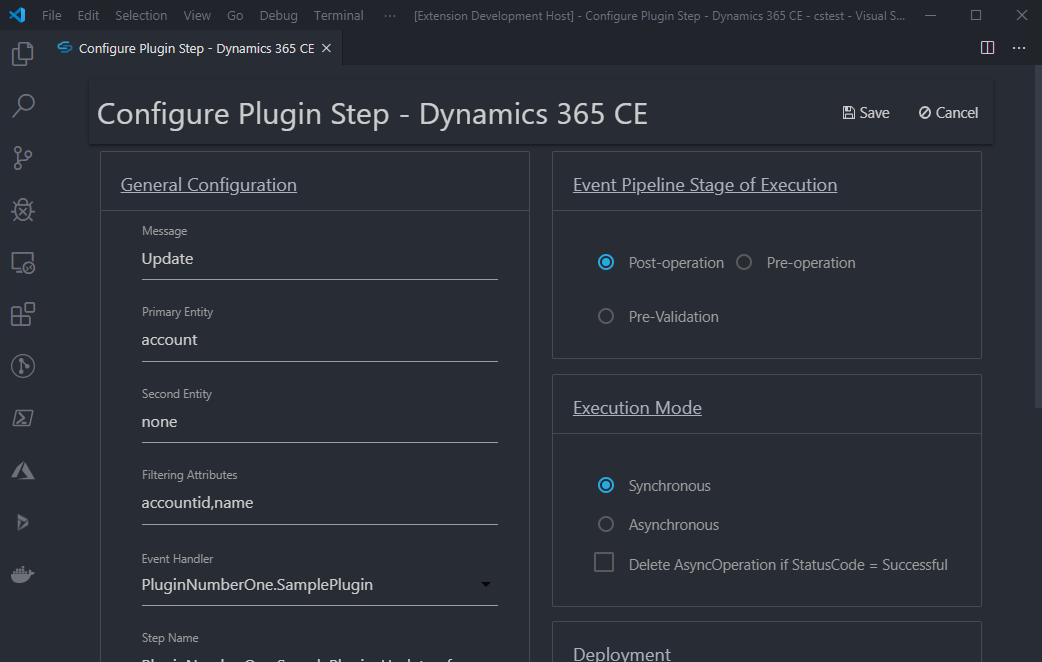
Note: Fields like message, primary entity and second entity will have auto complete
Modify or remove plugin steps
Plugin steps can be edited or deleted in CDS Explorer by:
- Open the CDS Explorer view by clicking the CDS for Code icon
- Expand the top level connection
- Expand the Organization
- Expand the Solutions folder
- Expand the target solution
- Expand the plugins folder
- Expand the target plugin assembly
- Expand the plugin namespace
- Mouse over the target plugin step and click the trash or pencil icon
The view for CDS Explorer will look similar to this:
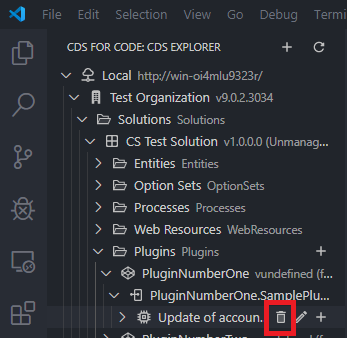
Plugin step images
Create plugin step images from CDS Explorer
Plugin steps images can be edited or deleted in CDS Explorer by:
- Open the CDS Explorer view by clicking the CDS for Code icon
- Expand the top level connection
- Expand the Organization
- Expand the Solutions folder
- Expand the target solution
- Expand the plugins folder
- Expand the target plugin assembly
- Expand the plugin namespace
- Mouse over the target plugin step and click plus icon
The view for CDS Explorer will look similar to this:
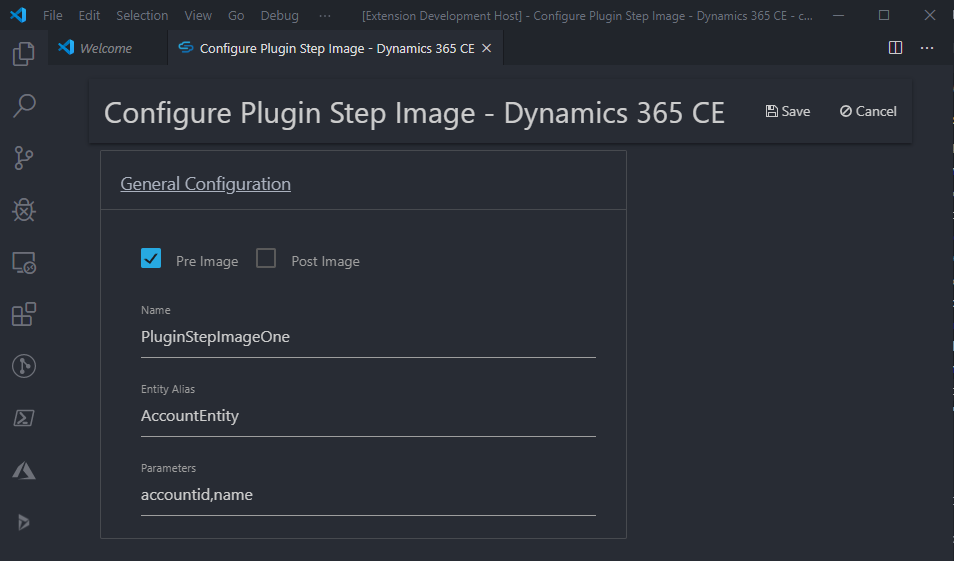
Modify or remove plugin step images
Plugin steps images can be edited or deleted in CDS Explorer by:
- Open the CDS Explorer view by clicking the CDS for Code icon
- Expand the top level connection
- Expand the Organization
- Expand the Solutions folder
- Expand the target solution
- Expand the plugins folder
- Expand the target plugin assembly
- Expand the plugin namespace
- Mouse over the target plugin step and click trash or pencil icon
What’s not included
- Managing plugin steps locally (persist to filesystem)
- Unpack plugin steps with solution update